Introduced by Anatoly Yakovenko in 2017, Solana helps eliminate scalability issues in the existing blockchains. Solana can process up to 710,000 transactions per second on a standard gigabit network, given that the transactions don’t exceed 176 bytes.
Solana is often hailed as the “Ethereum Killer.” In 2021, it established itself as a fast, secure, and censorship-resistant protocol: one of the rising stars in the blockchain space, with its Total Value Locked (TVL) from $144 million to $11 billion over the last nine months — just nine months. The credit for this 8X rise in TVL goes to the growing dApp and the quick adoption of Solana for token development.
Solana has great potential to transform the business world and is the fastest-growing ecosystem, compatible with global adoption. Furthermore, Solana has tremendous capabilities and many innovative features, such as smart contracts. In one of our recent articles, we discussed why one should consider building on Solana. Today, we will dive deep into how you can build and deploy smart contracts on the Solana blockchain. Let’s get started!
What Is Solana?
Shortest definition? Solana is a type of blockchain. It’s a decentralized blockchain ecosystem that was designed to help users avoid issues related to congestion and scalability that the existing blockchains couldn’t solve. Solana blockchain’s primary focus is to improve scalability by enabling higher transaction per second (TPS) and fast confirmation times.
Solana is an open-source project enabled by revolutionary technologies from Intel, Netscape, Google, and Qualcomm that support this blockchain ecosystem in maintaining its high-performing standards. In addition to offering fast, cheap, and scalable transactions. Solana supports the development of smart contracts with Rust, C++, and C programming languages.
The Architecture of Solana Smart Contracts
Solana has a slightly different smart contract model from traditional EVM-enabled blockchain contracts that combine code/logic and state it into a single contract deployed on-chain. Smart contracts on Solana remain in read-only or stateless mode and contain program logic only. As soon as you deploy a smart contract on Solana, it can easily be accessed by external accounts that interact with the main program to store data related to program interactions. It creates a significant difference between the state (accounts) and contract logic (programs), setting traditional EVM-enabled and Solana smart contracts apart.
Also, Solana doesn’t have the same accounts as other blockchains, such as Ethereum. Solana accounts store data (like wallet information), while Ethereum accounts act as references for users’ wallets. Another thing that sets Solana accounts apart is that Solana comes with a CLI and JSON RPC API that enhances dApps’ interaction with the Solana ecosystem. dApps can interact with Solana and programs on Solana by using existing SDKs.
Solana Smart Contracts — Development Workflow
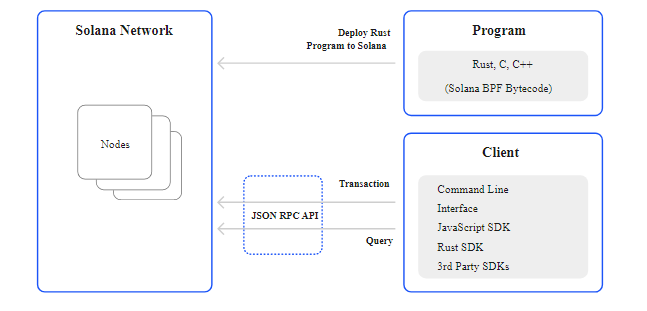
The Program on the right represents the development workflow using which you can create and deploy custom Rust, C, and C++ programs on the Solana blockchain. Once you have successfully deployed the programs, anyone who can code can use them. You need to write dApps with any available client SDKs in order to communicate with these programs. You also have to use the JSON RPC API under the hood.
Client, the second development workflow on the bottom right, allows you to write dApps for communicating with the deployed programs. These apps can create various applications like crypto wallets, decentralized exchanges, and more, and must submit transactions to these programs via client SDK. Program and Client work together to form a complete network of programs and dApps, capable of examining the blockchain.
Requirements for Creating a Smart Contract on Solana
To deploy a smart contract on Solana, you will need to install the following:
- NodeJS v14 or greater and NPM
- The latest stable Rust build
- Solana CLI v1.7.11 or later
- Git
How To Build a Smart Contract on Solana?
We will elaborate on how you can build smart contracts on Solana with a coding example. A little later, we will create and deploy a Solana smart contract called HelloWorld, written in Rust programming language. You can choose C or C++ too. Our program will print output to the console. Before we get to the development part, we must ensure that we have a functional Solana environment on Windows to make the work easier.
Set Up A Solana Development Environment
Most people find it confusing to run the smart contract code directly from Windows. You can set up an Ubuntu version of Window Subsystem for Linux (WSL) that will allow you to write the code in Windows. Later, you can compile the Rust smart contract into a .so file. You can set up the Solana development environment by following these commands:
apt upgradeapt updateapt install nodejsapt install npmapt install python3-pipcurl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | shsh -c "$(curl -sSfL https://release.solana.com/v1.5.7/install)"source $HOME/.cargo/envexport PATH="/root/.local/share/solana/install/active_release/bin:$PATH"export RUST_LOG=solana_runtime::system_instruction_processor=trace,solana_runtime::message_processor=debug,solana_bpf_loader=debug,solana_rbpf=debugsolana-test-validator --log
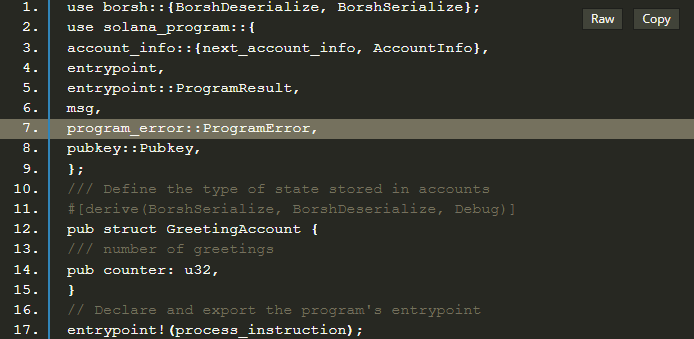
Once you have set up the Solana development environment, you need to test the Hello World application by running it. Here is how you can do it:
git clone https://github.com/solana-labs/example-helloworld.git cd example-HelloWorld/npm installnpm run build:program-rust
- Create a Solana Smart Contract in Rust
As mentioned earlier, we will create and deploy a Solana smart contract called HelloWorld. HelloWorld is a program in Rust or smart contract on Solana that will print our output to the console. It will also provide us with the number of times the HelloWorld program has been called for the given account. It will store the same number on-chain. Here is a breakdown of the code into separate sections.
The process_instruction Function
The first section of the program specifies some standard parameters for the Solana smart contract and helps us identify an entry point for the program (the ‘process_instruction’ function). In addition to the process_instruction function, this section uses Binary Object Representation Serializer for Hashing (BORSH) that serializes and deserializes parameters passing to and from the deployed program. You can set up the HelloWorld program by following these commands:
use borsh::{BorshDeserialize, BorshSerialize};
use solana_program::{
account_info::{next_account_info, AccountInfo},
entrypoint,
entrypoint::ProgramResult,
msg,
program_error::ProgramError,
pubkey::Pubkey,
};
/// Define the type of state stored in accounts
#[derive(BorshSerialize, BorshDeserialize, Debug)]
pub struct GreetingAccount {
/// number of greetings
pub counter: u32,
}
// Declare and export the program's entrypoint
entrypoint!(process_instruction);
Now, you will notice that the process_instruction function accepts the program_id (a public key where the program is being deployed) and the accountInfo (the account used to say hello to).
pub fn process_instruction(
program_id: &Pubkey, // Public key of the account the hello world program was loaded into
accounts: &[AccountInfo], // The account to say hello to
_instruction_data: &[u8], // Ignored, all helloworld instructions are hellos
The ProgramResult Function
The ProgramResult function stores the main logic of the program. In our case, it will print a message first and then choose the desired account from the “accounts.” Please note that we are using only one account.
-> ProgramResult {
msg!("Hello World Rust program entrypoint")
// Iterating accounts is safer than indexing
let accounts_iter = &mut accounts.iter();
// Get the account to say hello to
let account = next_account_info(accounts_iter)?;
After choosing an account, the program will check if it has permission to change data for the chosen account.
// The account must be owned by the program to modify its data
if account.owner != program_id {
msg!("Greeted account does not have the correct program id");
return Err(ProgramError::IncorrectProgramId);
}
The Result
At last, the function reverts the stored number of the chosen account, increases the value by one, records the result, and displays it with a message.
// Increment and store the number of times the account has been greeted
let mut greeting_account = GreetingAccount::try_from_slice(&account.data.borrow())?;
greeting_account.counter += 1;
greeting_account.serialize(&mut &mut account.data.borrow_mut()[..])?;
msg!("Greeted {} time(s)!", greeting_account.counter);
Ok(())
Deploy the Smart Contract
To deploy the smart contract on Solana or the program, you need to clone the repository first.
git clone https://github.com/solana-labs/example-helloworld
cd example-HelloWorld
Once you are done cloning the repository, set the current environment to Devnet — the test network for Solana developers where they can write smart contracts.
solana config set --url https://api.devnet.solana.com
Now, you need to create a new keypair so that you can interact with the deployed program or smart contract on the Solana Devnet. You can generate a new keypair using the solana-keygen new command.
Solana-keygen new --force
After creating an account, use the airdrop program to obtain the required SOL tokens. Please keep in mind that it will require you to do some imports for the smart contract deployment. Use this command:
Solana airdrop 5
At this point, you’re good to build the HelloWorld program. Use the below command to build it.
npm run build:program-rust
Deploy the program to Devnet once you have built it. The previous command’s output will provide you with the command to run the program, looking similar to this:
Solana program deploy dist/program/HelloWorld.so
That’s all. And voilá! You have successfully deployed the HelloWorld program to Devnet. If you want to authenticate the program, you can do it on the Solana Devnet explorer.
Solana Blockchain Development With Rather Labs
There is no doubt that Solana is a high-performing and one of the fastest-developing blockchain ecosystems, which offers superior scalability. Adding more to the list of Solana features, it supports the development of various types of smart contracts that power dApps, such as NFT marketplaces, P2P lending systems, Wallets, DEXs, and so on.
At Rather Labs, we have a group of expert engineers with years of experience in Rust and Solana. We have successfully deployed many blockchain projects, including Hatom, the first lending protocol in ELROND, and NRC-721, the first NFT standard in Nervos Blockchain. Our blockchain experts are well-versed in every type of blockchain and ready to work on your next big project. We offer a wide range of end-to-end Solana blockchain development services, including:
- Staff augmentation
- Smart contract development and audit
- DeFi development
- Blockchain consulting
- dApp development
- NFT marketplace development
- SPL token development
- Exchange integration
- Wallet development
- Node development and maintenance
We are here to help you with our dApps development services that exceed expectations and help you launch user-friendly decentralized applications powered by Solana's high scalability and speed. Get in touch with us to know more.
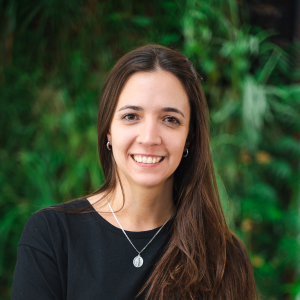